Co-Authored By:
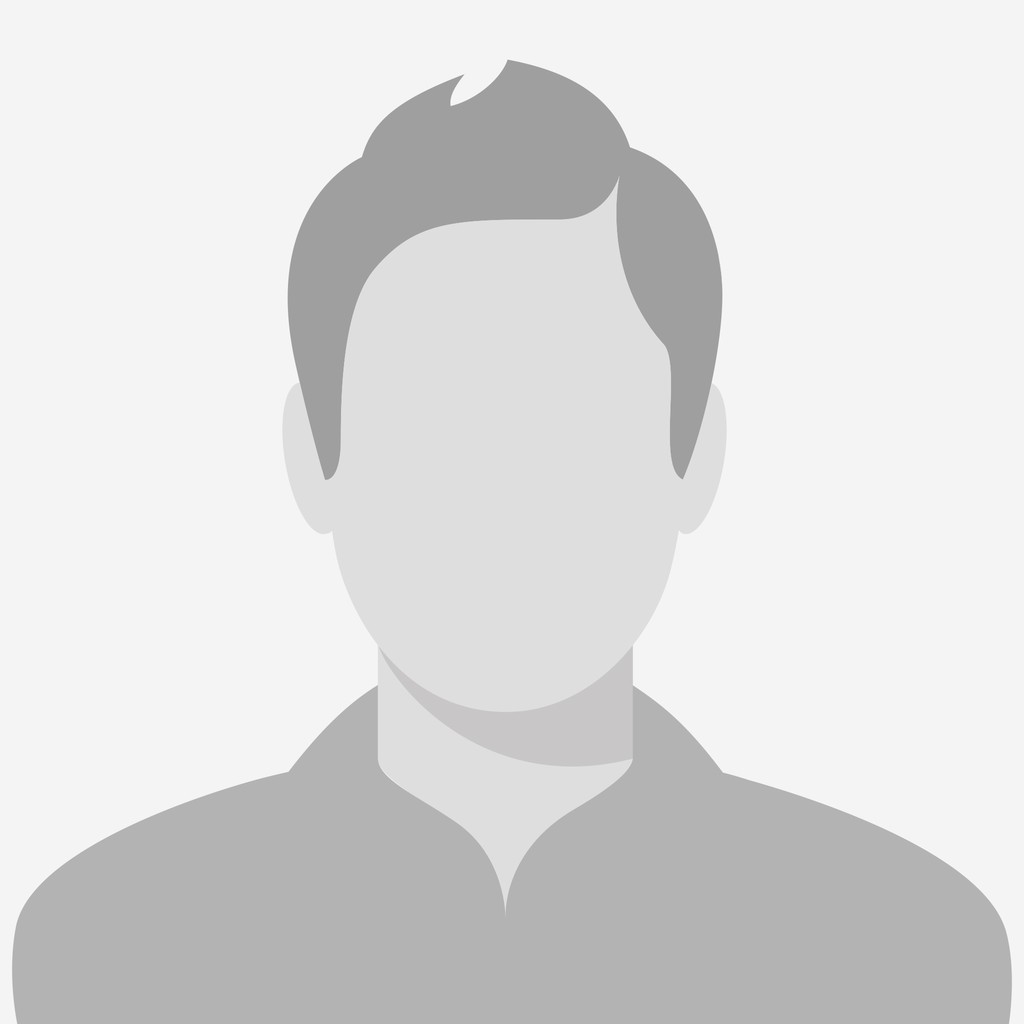
Asked by: Kia Fornell
technology and computing programming languagesCan we import interface in Java?
Also question is, how do interfaces work in Java?
Java uses Interface to implement multiple inheritance. A Java class can implement multiple Java Interfaces. All methods in an interface are implicitly public and abstract. To use an interface in your class, append the keyword "implements" after your class name followed by the interface name.
In respect to this, why interfaces are used in Java?
It is used to achieve total abstraction. Since java does not support multiple inheritance in case of class, but by using interface it can achieve multiple inheritance . It is also used to achieve loose coupling.
An interface cannot contain instance fields. The only fields that can appear in an interface must be declared both static and final. An interface is not extended by a class; it is implemented by a class. An interface can extend multiple interfaces.