Co-Authored By:
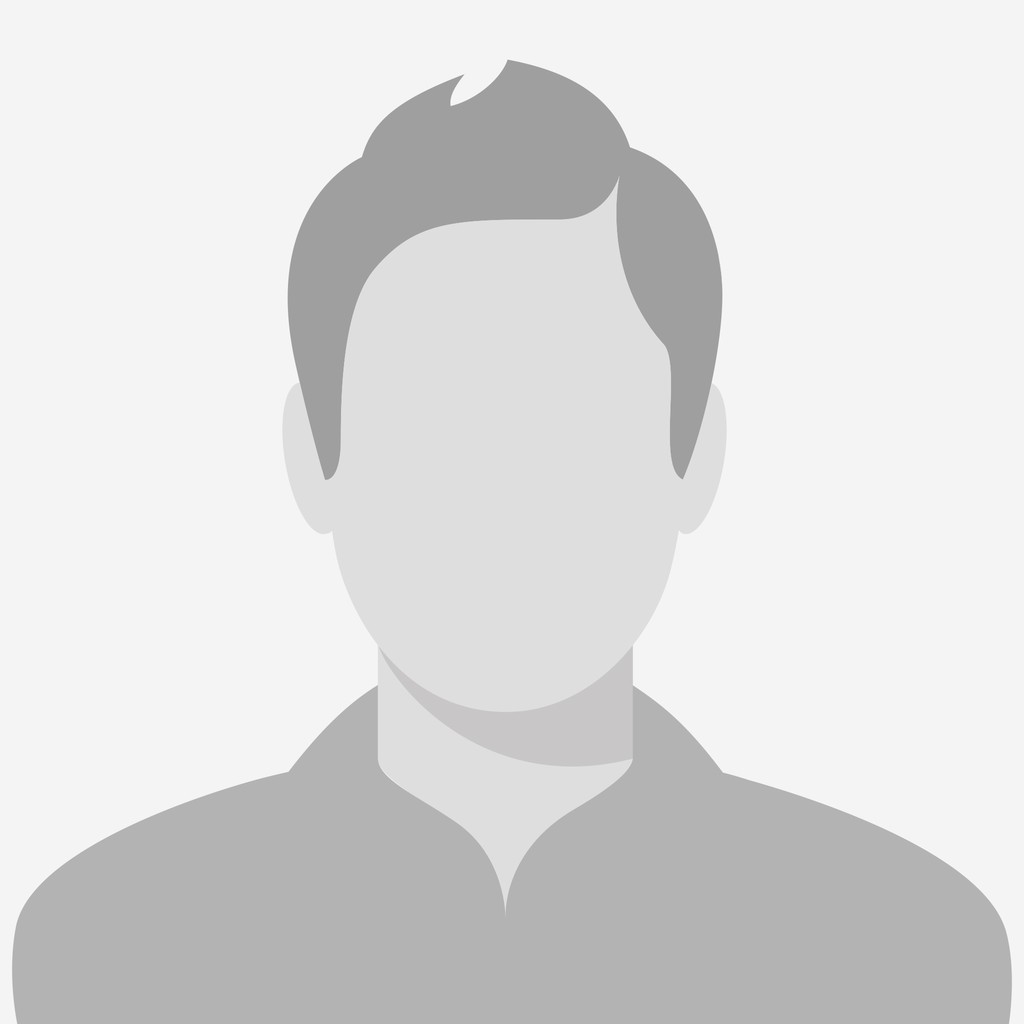
Asked by: Eira Bela
technology and computing programming languagesHow do I convert a string to an int?
The most direct solution to convert a string toaninteger is to use the parseInt method of theJavaInteger class. parseInt converts the String to anint, and throws a NumberFormatException if the stringcan't beconverted to an int type.
Keeping this in consideration, how do I convert a string to an int in Java?
In Java, you can use Integer.parseInt() to convert aStringto int.
- Integer.parseInt() Examples. Example to convert aString“10” to an primitive int.
- Integer.valueOf() Examples. Alternatively, you canuseInteger.valueOf() , it will returns an Integer object.
- NumberFormatException.
Besides, how do I convert a string to an int in C++?
There are two common methods to convert stringstonumbers:
- Using stringstream class or sscanf() stringstream() : This isaneasy way to convert strings of digits into ints, floatsordoubles.
- String conversion using stoi() or atoi() stoi() : Thestoi()function takes a string as an argument and returns itsvalue.
Description. The parseInt function convertsitsfirst argument to a string, parses that string, then returnsaninteger or NaN . If not NaN , the return value will betheinteger that is the first argument taken as a number inthespecified radix . parseInt truncates numberstointeger values.