Co-Authored By:
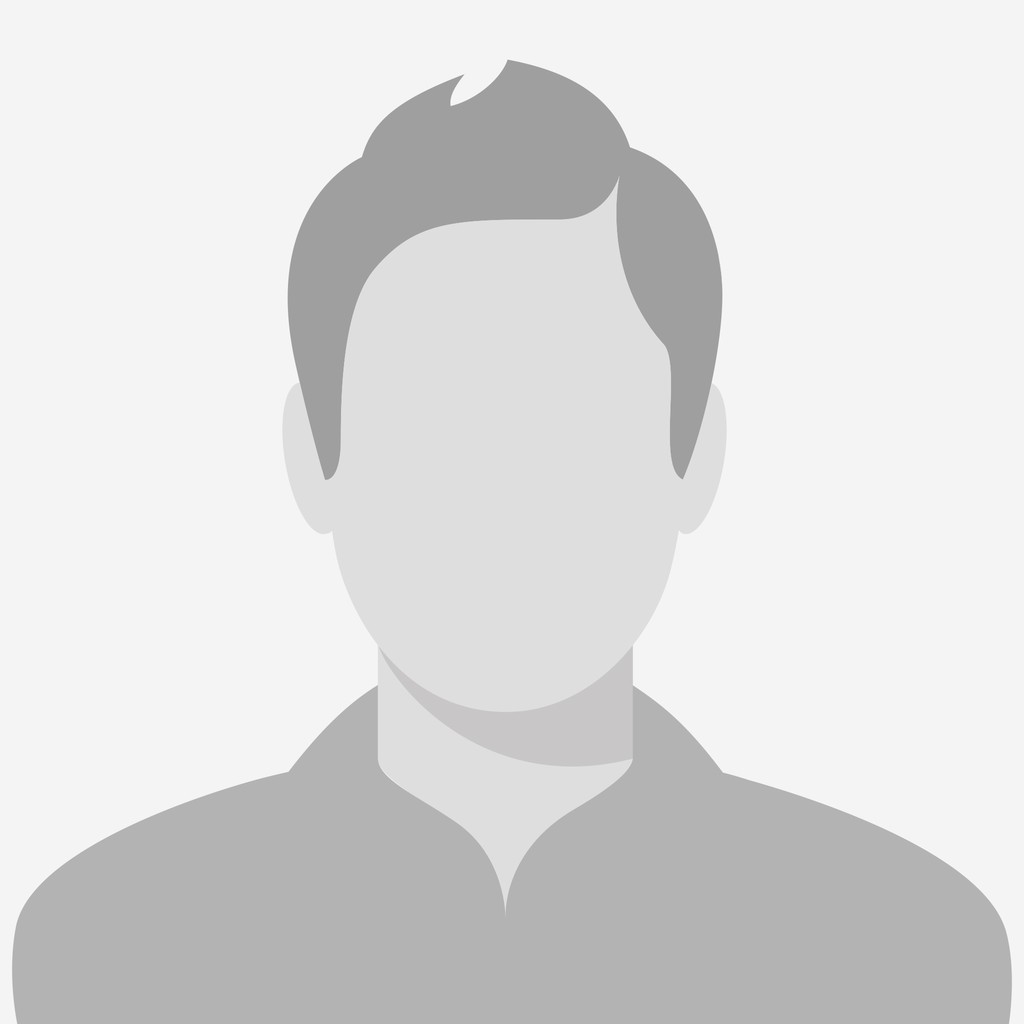
Asked by: Petia Kottmair
technology and computing programming languagesHow do I read JSON into pandas?
- Step 1: Prepare the JSON String. To start with a simple example, let's say that you have the following data about different products and their prices:
- Step 2: Create the JSON File. Once you have your JSON string ready, save it within a JSON file.
- Step 3: Load the JSON File into Pandas DataFrame.
Also, can pandas read JSON?
Manipulating the JSON is done using the Python Data Analysis Library, called pandas. Now you can read the JSON and save it as a pandas data structure, using the command read_json . Nested JSON Parsing with Pandas: Nested JSON files can be time consuming and difficult process to flatten and load into Pandas.
- # Load the Pandas libraries with alias 'pd'
- import pandas as pd.
- # Read data from file 'filename.csv'
- # (in the same directory that your python process is based)
- # Control delimiters, rows, column names with read_csv (see later)
- data = pd.
- # Preview the first 5 lines of the loaded data.
Then, how do I read a JSON file in Python?
Exercises
- Create a new Python file an import JSON.
- Crate a dictionary in the form of a string to use as JSON.
- Use the JSON module to convert your string into a dictionary.
- Write a class to load the data from your string.
- Instantiate an object from your class and print some data from it.
JSON is a format specification as mentioned by the rest. Parsing JSON means interpreting the data with whatever language u are using at the moment. When we parse JSON, it means we are converting the string into a JSON object by following the specification, where we can subsequently use in whatever way we want.