Co-Authored By:
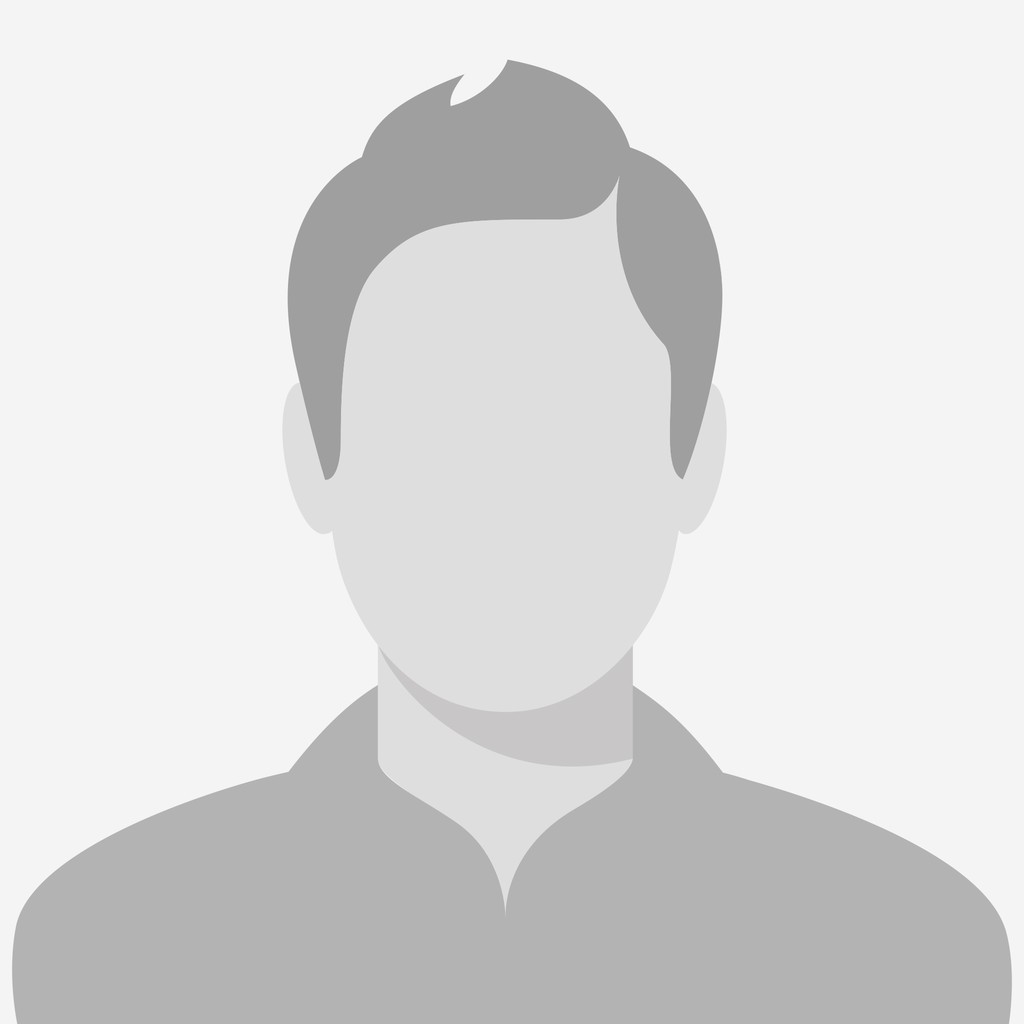
Asked by: Jorgelina Meredith
technology and computing programming languagesHow do you avoid array index out of bound exception?
Also know, what is array index out of bound exception?
The array index out of bounds error is a special case of the buffer overflow error. It occurs when the index used to address array items exceeds the allowed value. It's the area outside the array bounds which is being addressed, that's why this situation is considered a case of undefined behavior.
- name.
- When accessing the contents of an array, position starts from 0.
- When you loop, since i can be less than or equal to name.
Correspondingly, how do you handle an array out of bound exception in Java?
The index of an array is an integer value that has value in interval [0, n-1], where n is the size of the array. If a request for a negative or an index greater than or equal to size of array is made, then the JAVA throws a ArrayIndexOutOfBounds Exception. This is unlike C/C++ where no index of bound check is done.
lang. StringIndexOutOfBoundsException if beginIndex is negative, or larger than the length of the string. This method returns a sub-string that begins with the character at the specified index and extends until the character at endIndex-1 index. It throws an java.