Co-Authored By:
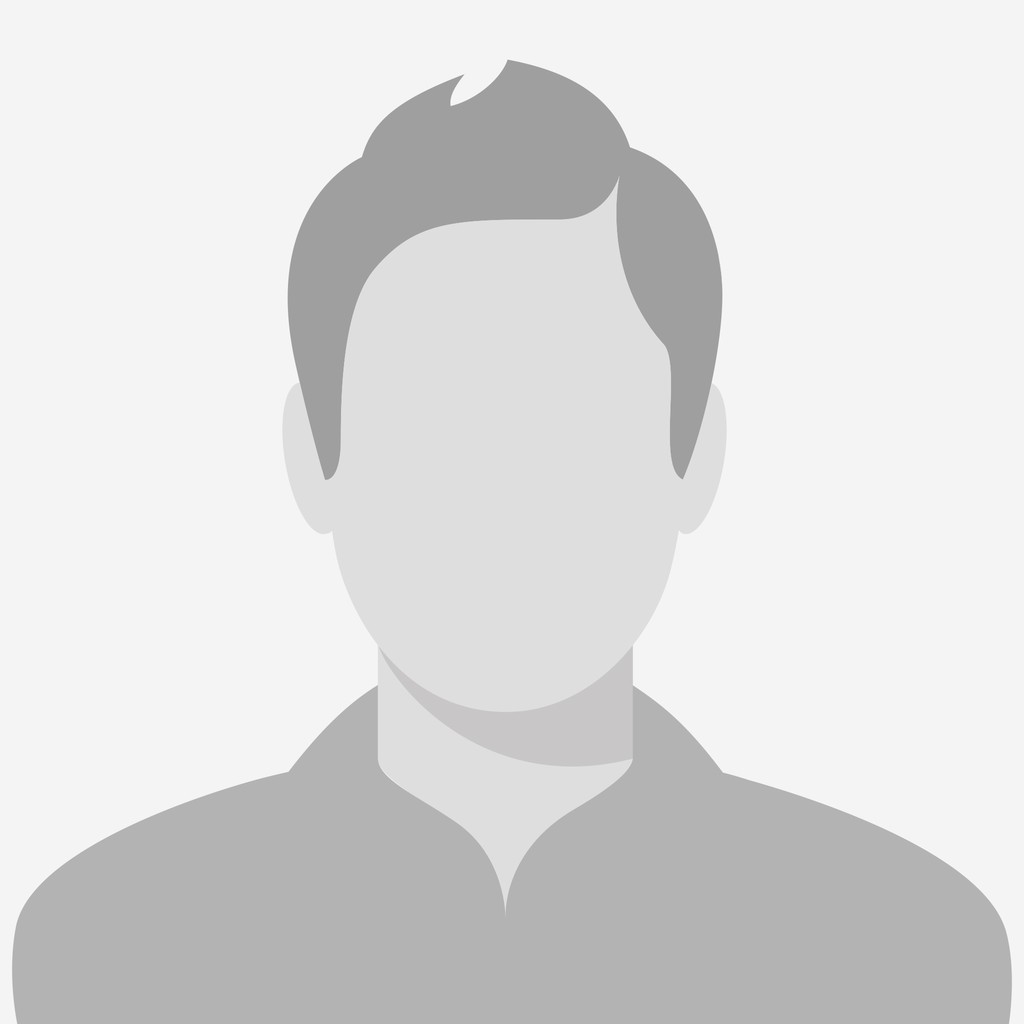
Asked by: Niria Astarbe
technology and computing programming languagesWhat causes an infinite loop in Java?
Keeping this in consideration, what is an infinite loop explain with an example?
An infinite loop (sometimes called an endlessloop ) is a piece of coding that lacks a functional exit sothat it repeats indefinitely. Usually, an infinite loopresults from a programming error - for example, where theconditions for exit are incorrectly written.
Secondly, how do you stop infinite loops?
Here are some notes to bear in mind to help you avoidinfinite loops:
- The statements in the for() block should never change the valueof the loop counter variable.
- In while() and do…while() loops, make sure you have atleast one statement within the loop that changes the value of thecomparison variable.
An infinite loop (or endless loop)is a sequence of instructions in a computer program whichloops endlessly, either due to the loop having noterminating condition, having one that can never be met, orone that causes the loop to start over. Busy wait loopsare also sometimes called "infinite loops".