Co-Authored By:
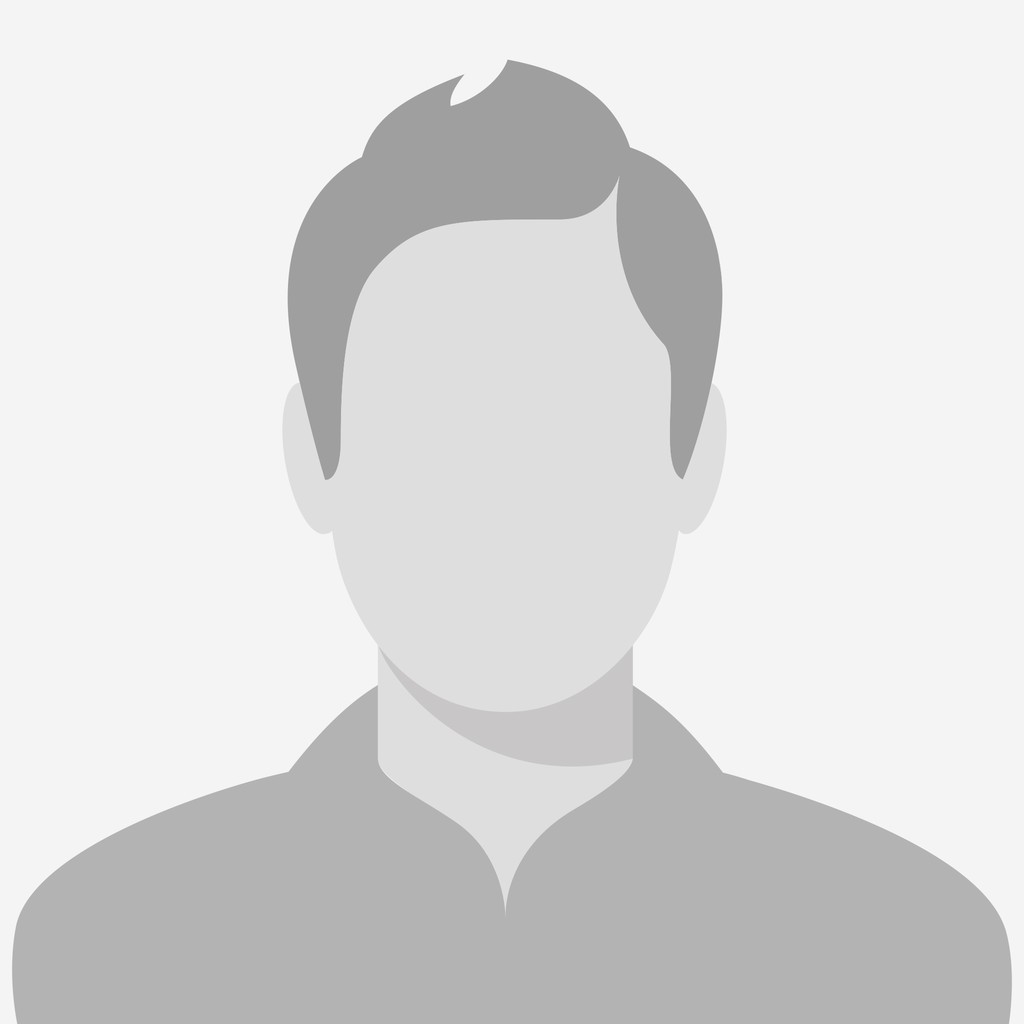
Asked by: Soiartze Bret
technology and computing programming languagesWhat is circular doubly linked list?
Thereof, what is doubly linked list explain?
A doubly linked list is a kind of linkedlist with a link to the previous node as well as a datapoint and the link to the next node in the list aswith singly linked list. A sentinel or null node indicatesthe end of the list. Doubly linked lists aretypically implemented in pseudocode in computer sciencetextbooks.
Also to know, what is circular linked list?
A circular linked list is a sequence of elementsin which every element has a link to its next element in thesequence and the last element has a link to the firstelement. That means circular linked list is similar to thesingle linked list except that the last node points to thefirst node in the list.
a doubly linked list needs more operations whileinserting or deleting and it needs more space (to store theextra pointer). A doubly linked list can be traversed inboth directions (forward and backward). A singly linked listcan only be traversed in one direction.