Co-Authored By:
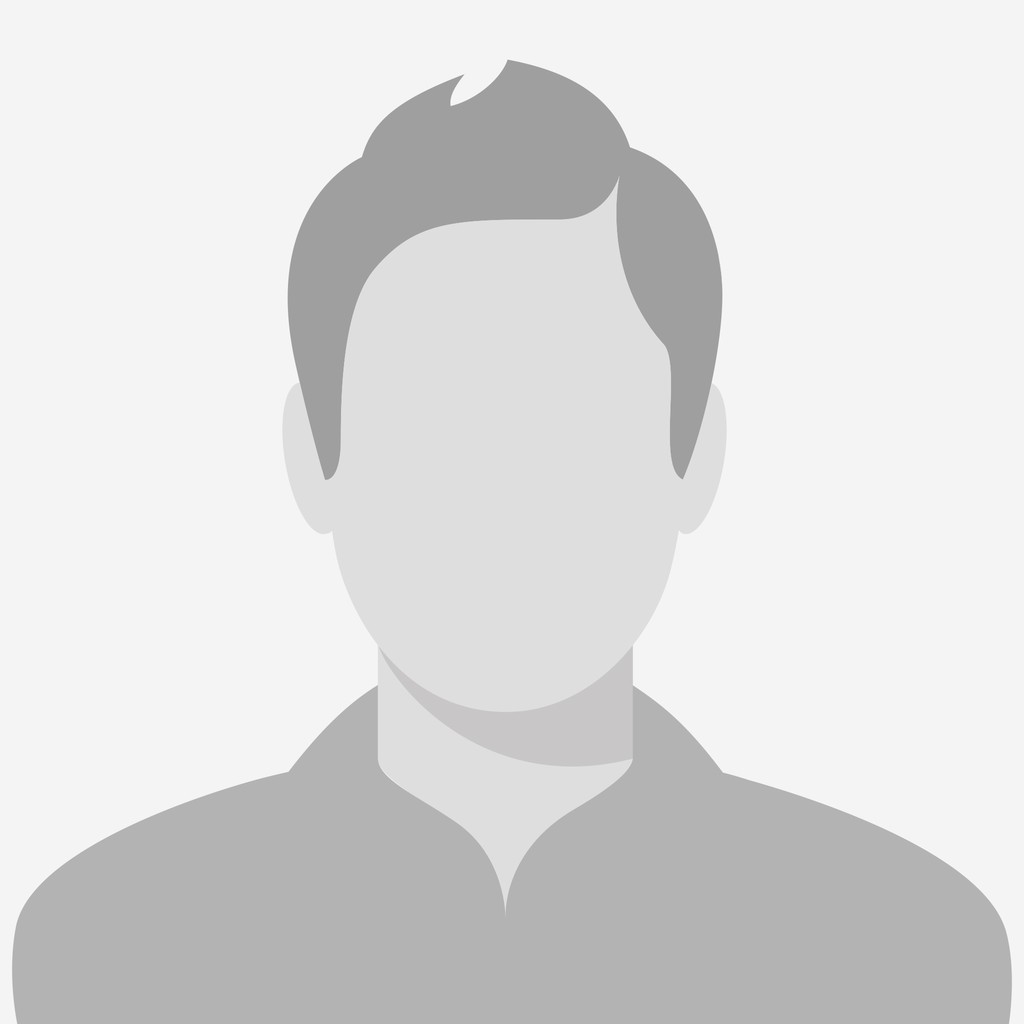
Asked by: Abdeslem Chindris
technology and computing programming languagesWhat is type casting in Python?
Likewise, people ask, what is type casting with example?
An example of typecasting is convertinganinteger to a string. In order to round to the nearest value,adding0.5 to the floating point number and then typecastingit toan integer will produce an accurate result. Forexample, inthe function below, both 2.75 and 3.25 will getrounded to3.
what is type () in Python?
Python | type() function type() method returns class type oftheargument(object) passed as parameter. If singleargumenttype(obj) is passed, it returns the type ofgivenobject. If three arguments type(name, bases, dict)ispassed, it returns a new type object.
Type casting refers to changing anvariableof one data type into another. For instance,if you assign aninteger value to a floating-point variable,the compiler willconvert the int to a float. Casting allowsyou to make thistype conversion explicit, or to force itwhen it wouldn'tnormally happen.